Hello world with LibGDX
libGDX is a cross-platform Java game development library. It supports Windows, Gnu/Linux, Android, iOS, Mac, and web. The desktop variations use the Lightweight Java Game Library (LWJGL), with OpenGL support. There are already good comprehensive tutorials out there, so this article will only present the “Hello World” example with a small animation.
As opposed to basic OpenGL libraries, LibGDX and LWJGL offer a complete framework for creating a game, animations or interactions. To lower the bar to entry, the ApplicationListener interface has the common methods, most important the render() method, which is automatically called in a loop.
In the example below, a rotation matrix is defined and set, followed by drawing of the words “Hello World”. Because this method is called in a loop, the angle variable will update, and thus set a new rotation matrix every time. Similarly, the color is updated for each frame. The effect is a continuous never-ending animation.
@Override
public void render() {
Gdx.gl.glClearColor(1, 1, 1, 1);
Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT);
Matrix4 rotate = new Matrix4();
rotate.rotate(new Vector3(0, 0, 1), angle--);
rotate.trn(200, 200, 0);
batch.setTransformMatrix(rotate);
batch.begin();
font.setColor(new Color(color++ % 255));
font.draw(batch, "Hello World", -40, 0);
batch.end();
}
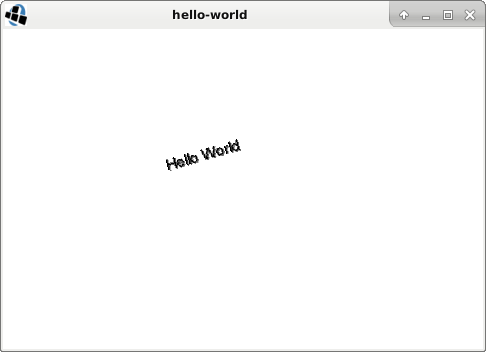
There are multiple GDX packages, for different platforms and features, and for this example the following three are needed from the Maven Central repository. Be sure to include the natives-desktop version annotation on the gdx-platform package. For other platforms, e.g. Android, other packages are needed.
repositories {
mavenCentral()
}
dependencies {
compile 'com.badlogicgames.gdx:gdx:1.9.6'
compile 'com.badlogicgames.gdx:gdx-platform:1.9.6:natives-desktop'
compile 'com.badlogicgames.gdx:gdx-backend-lwjgl:1.9.6'
}
Here is the full code, as a stand-alone application.
package com.rememberjava.graphics;
import com.badlogic.gdx.ApplicationListener;
import com.badlogic.gdx.Gdx;
import com.badlogic.gdx.backends.lwjgl.LwjglApplication;
import com.badlogic.gdx.backends.lwjgl.LwjglApplicationConfiguration;
import com.badlogic.gdx.graphics.Color;
import com.badlogic.gdx.graphics.GL20;
import com.badlogic.gdx.graphics.g2d.BitmapFont;
import com.badlogic.gdx.graphics.g2d.SpriteBatch;
import com.badlogic.gdx.math.Matrix4;
import com.badlogic.gdx.math.Vector3;
public class LibGdxHelloWold implements ApplicationListener {
private SpriteBatch batch;
private BitmapFont font;
private float angle;
private int color;
public static void main(String[] args) {
LwjglApplicationConfiguration cfg = new LwjglApplicationConfiguration();
cfg.title = "hello-world";
cfg.width = 480;
cfg.height = 320;
new LwjglApplication(new LibGdxHelloWold(), cfg);
}
@Override
public void create() {
batch = new SpriteBatch();
font = new BitmapFont();
font.setColor(Color.RED);
}
@Override
public void dispose() {
batch.dispose();
font.dispose();
}
@Override
public void render() {
Gdx.gl.glClearColor(1, 1, 1, 1);
Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT);
Matrix4 rotate = new Matrix4();
rotate.rotate(new Vector3(0, 0, 1), angle--);
rotate.trn(200, 200, 0);
batch.setTransformMatrix(rotate);
batch.begin();
font.setColor(new Color(color++ % 255));
font.draw(batch, "Hello World", -40, 0);
batch.end();
}
@Override
public void resize(int width, int height) {}
@Override
public void pause() {}
@Override
public void resume() {}
}